How to Spawn Objects in Unity
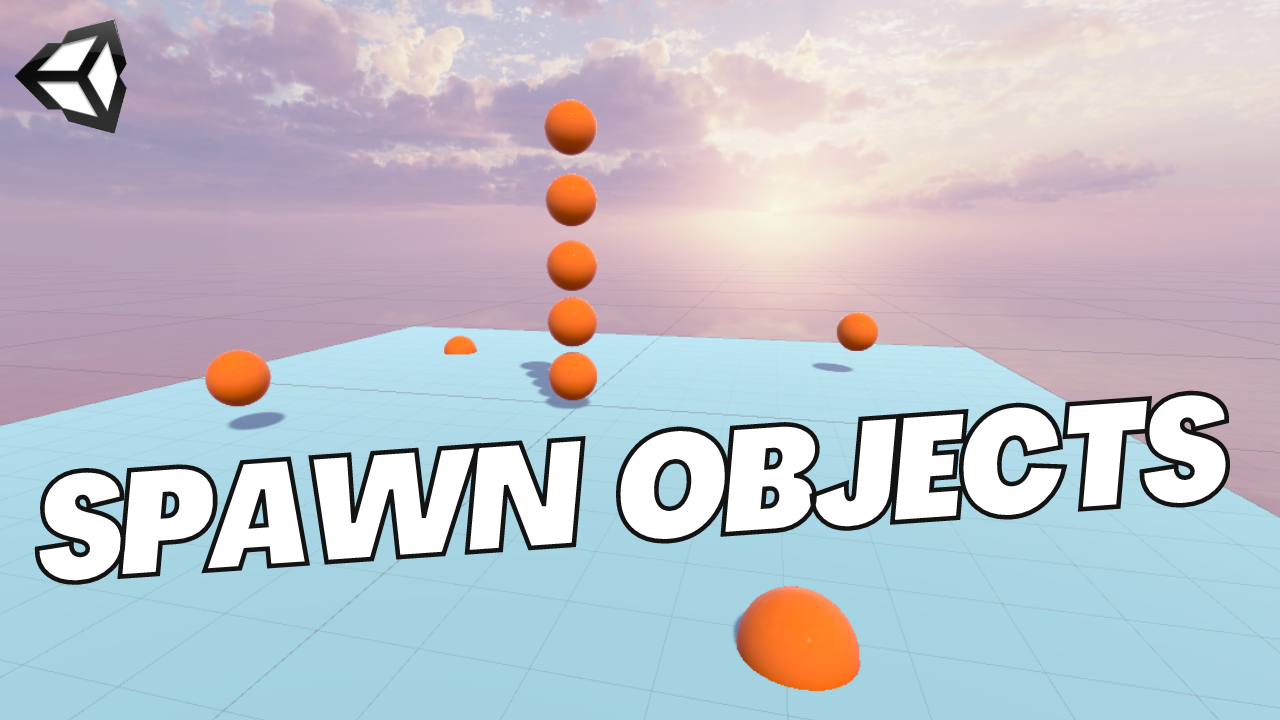
If you're learning game development, at some point you're going to want to learn how to spawn objects in Unity using logic in C#. Spawning game objects is an important part of game development because you'll want the ability to be able to spawn enemies, vegetation, coins, and much more.
Scene Setup
If we want to spawn an object, we must first have an object to spawn. Let's create a Prefab so we can use this game object over and over again.
Anything can be a Prefab, but in this case I've created a simple sphere that I'll drag and drop into the Asset folder window.
Setting up the Logic
With the game object we want to spawn created, now it's time to set up our logic. Let's add a new game object into the scene to hold this logic. On the new game object, add a new script. I've named mine "Spawner."
Now heading over to Visual Studio, we need to tell Unity which object we want to spawn, so I'm going to create a public gameobject to hold the object we want to spawn. (We will drag and drop our Prefab into this slot later).
Next, for the actual logic we're going to create a function. It has to be public since we want it attached to a button in the Editor, and it's going to be void since we're not returning any value. The logic is pretty simple, it's just Instantiate, and then passing through the variable that we just created, mySphere.
But now, our logic doesn't know what mySphere is in the context of Unity, so this is where our prefab comes in. We can just drag and drop our sphere prefab into the slot we created, and we're good to go there.
Now to actually test this logic, we need to create a button to call it, which will require a canvas object. After we've created the UI Canvas we can right click > UI > button. If you get a prompt to import TextMeshPro Essentials, go ahead and do that.
Right now, the logic just kind of exists in the void and is waiting to be used.
There are multiple ways to test your logic, I see a lot of other YouTubers will use the space key to test, but using a button is just another way.
To hook up our logic, we'll go to our button element and find our way down to the On Click section, and click on the plus sign. In the first section that pops up, we need to drag and drop the spawner game object into this slot, since this is where the logic lives. Once we do that, since our function is public it will pop up as an option for us to select.
And boom, we have a working button.
If we navigate back to the main part of the scene, we can just delete this sphere since we already have our logic done. Now if we press play, we can see that every time you press the button, a new sphere appears. But chances are, you probably don't want something to spawn in the same place over and over again, and if that's the case, follow me to the next video/article where I'll show you how to spawn objects at random places.